My favorite new development in CSS3 is the addition of keyframe animation. Webkit was the pioneer and first implementer of keyframe animation. For a couple years it was only available in Safari and Chrome, but as of June 21st of this year, it is included now in Firefox, which makes it a very viable for mainstream use, especially since animation typically isn't mission-critical.
The word "animation" typically calls up thoughts of Toy Story and Rugrats, but keyframe animation wasn't designed for the purpose of cinematic animation, it's meant more for interface enhancements. That hasn't stopped some people from trying anyway.
It looks complicated, but it's actually not very difficult. You start by defining the keyframes, which are the extreme points on either end of any movement. Say you find some reason to animate a block of text from white to black. You'd define one keyframe as white, the other as black and the browser fills the frames in between the two to make a smooth transition. You start with the vendor prefix—I'll be using webkit, but just change any instance of "webkit" to "moz" for Firefox. Insert the "keyframes" keyword and then name it. For each keyframe, specify which CSS properties you want to change. Color, size, position, rounded corners, borders, rotation, opacity, etc. Anything goes. Here's the code:
@-webkit-keyframes colorchange{
from{
color: #fff;
}
to{
color: #000;
}
}
To apply it to an element, use the animation shorthand property. This example shows the only two required values, the animation name and duration:
#someelement{
-webkit-animation: colorchange 8s;
}
The Nitty Gritty
So, those are the basics. Let me give you a few more tools to spice your animations up a smidge. You can start by getting more specific with your keyframes. Using from and to is nice for two-state animations, but you can go a lot further than that. Using percentage values instead will let you add many more frames in between. From and to can be substituted with 0% and 100%, respectively. Add more frames in between with other percentage values. Most browsers even support decimal percentages, though there don't seem to be any clear answers as to which place they round to. You're probably safe with hundredths. This means you could theoretically use up to 10,000 keyframes!
There are also some handy keywords you can use in the CSS property to gain more control over your animation. We're going to stick with the shorthand property for simplicity's sake.
- Adding another seconds value after the length value will define the animation delay value so you can prevent your animation from beginning right away. Both of the time values can be defined with decimals as well, by the way.
- You can define the number of iterations by adding another number to the list of values. Decimal numbers here will do a partial iteration at the end. Or you can use the "infinite" keyword and it will repeat forever.
- Easing keywords can alter the acceleration of your animation. Ease-in gets progressively faster. Ease-out gets progressively slower. Ease-in-out is slow on both ends. Linear (the default) is the same speed all the way through.
- Add the "alternate" keyword, and your animation will play through forwards and then repeat backwards. This is useful for infinitely repeating animations so there isn't an abrupt jolt when the animation restarts. Throbbing, glowing and pulsing are easy to achieve with alternating animations.
One little known secret: you can apply multiple animations to the same element. A little bug in webkit browsers (Safari and Chrome) will only accept the first timing function (easing) for all of the animations assigned to one element. This should be fixed soon—it's already fixed in the webkit nightly build. Just separate the declarations by commas like this:
-webkit-animation: colorchange 5s infinite alternate,
slideleft 10s 4s 6 alternate;
Spinning Example:
#star{
-webkit-animation: spinner 3s infinite linear;
}
@-webkit-keyframes spinner{
0%{
-webkit-transform: rotate(0deg);
}
100%{
-webkit-transform: rotate(360deg);
}
}
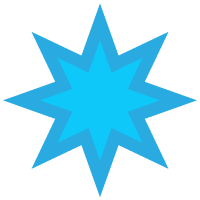
Wave Example:
#reddot{
position: absolute;
-webkit-animation: upanddown 1s infinite ease-in-out alternate, ltor 8s infinite linear;
}
@-webkit-keyframes ltor{
from{
left: 0%;
}
to{
left: 100%;
opacity: 0;
}
}
@-webkit-keyframes upanddown{
from {
top: 10px;
}
to{
top: 200px;
}
}
